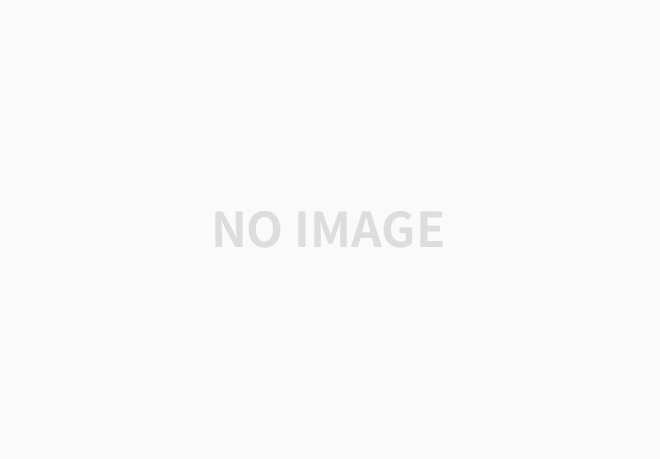
강의 - www.fastcampus.co.kr/dev_online_iosapp
iOS 앱 개발 올인원 패키지 Online. | 패스트캠퍼스
차근차근 따라하다 보면 어느새 나만의 iOS 앱을 개발할 수 있게 됩니다.
www.fastcampus.co.kr
깃허브 - github.com/FreeDeveloper97/iOS-Study-FastCompus
FreeDeveloper97/iOS-Study-FastCompus
Contribute to FreeDeveloper97/iOS-Study-FastCompus development by creating an account on GitHub.
github.com
05. 스위프트 Function and Optional
" function "
" function "
/* function */
func printName() {
print("--> My name is Jason")
}
printName()
// 1. 성, 이름을 받아서 fullname을 출력하는 함수 만들기
func printFullname(firstName: String, lastName: String) {
print("--> \(firstName) \(lastName)")
}
printFullname(firstName: "Jason", lastName: "Lee")
" function - parameter "
parameter : 함수에서 변수를 받는 인자역할
// param 1개
// 숫자를 받아서 10을 곱해서 출력한다
func printMutipleOfTen(value: Int) {
print("\(value) * 10 = \(value * 10)")
}
printMutipleOfTen(value: 5)
// param 2개
// 물건값과 개수를 받아서 전체 금액을 출력하는 함수
func printTotalPrice(price: Int, count: Int) {
print("Total price: \(price * count)")
}
printTotalPrice(price: 1500, count: 5)
" function - external parameter "
extarnal paramemter name : 함수를 사용시에 변수전달을 파라미터명 대신 다른이름으로 전달하는 방법
1. 다른 이름을 사용하여 전달하는 경우 : 파라미터 앞에 적으면 된다
// external parameter names 설정방법
func printTotalPrice(가격 price: Int, 개수 count: Int) {
print("Total price: \(price * count)")
}
printTotalPrice(가격: 1500, 개수: 5)
2. 변수값만으로 전달하는 경우 : 파라미터 앞에 _ 사용하면 된다
func printTotalPrice(_ price: Int, _ count: Int) {
print("Total price: \(price * count)")
}
printTotalPrice(1500, 5)
// 2. 1번에서 만든 함수인데, 파라미터 이름을 제거하고 fullname 출력하는 함수 만들기
func printFullname(_ firstName: String, _ lastName: String) {
print("--> \(firstName) \(lastName)")
}
printFullname("Jsson", "Lee")
" function - default value "
default value : 함수 사용시 입력값이 없더라도 기본값을 설정하여 사용하는 방법
-> 변수타입 뒤에 기본값을 설정하면 된다
// default value 설정방법
func printTotalPriceWithDefaultValue(price: Int = 1500, count: Int) {
print("Total price: \(price * count)")
}
printTotalPriceWithDefaultValue(count: 5)
printTotalPriceWithDefaultValue(price: 2000, count: 5)
" function - return "
반환값이 있는 function
-> 반환타입을 적으면 된다
// 반환값이 있는 function
func totalPrice(price: Int, count: Int) -> Int {
let totalPrice = price * count
return totalPrice
}
let calcupatedPrice = totalPrice(price: 10000, count: 77)
// 3. 성, 이름을 받아서 fullname return 하는 함수 만들기
func fullname(firstName: String, lastName: String) -> String {
return "\(firstName) \(lastName)"
}
let name = fullname(firstName: "Jason", lastName: "Lee")
name
" function - overload "
overload : 같은 함수명, 다른 함수내용을 뜻
-> 즉 비슷한 역할을 하나 파라미터 타입, 또는 반환타입이 다른 경우
// 다양한 반환 내용이 필요할 때 overload를 사용한다
func printTotalPrice(price: Int, count: Int) {
print("Total Price: \(price * count)")
}
/* overload : 같은 이름, 다른 행동 */
func printTotalPrice(가격: Double, 개수: Double) {
print("총 가격은 \(가격 * 개수)")
}
" function - inout parameter "
parameter는 변하지 않는 const 값인데 변수로 사용하기 위한 방법
-> 파라미터 변수타입 앞에 inout 붙이고, 함수 사용시 &붙여서 변수를 넣는다
/* in out parameter */
/* parameter 는 변하지 않는 let 변수이다 */
/* 변수로 사용하려면 inout 키워드가 필요하다 */
/* 또한 함수를 사용시에 변수에 & 기호가 필요하다 */
var value = 3
func incrementAndPrint(_ value: inout Int) {
value += 1
print(value)
}
incrementAndPrint(&value)
" function - function as parameter "
함수를 변수, 또는 parameter로 사용하는 방법
-> 특정 함수에 파라미터로 함수자체를 전달이 가능하다!
다만, 전달되는 함수의 입력, 반환 타입이 동일한 함수들끼리 전달이 가능하다!
/* function as a parameter : 함수를 파라미터로 넘기는 방법 */
/* 함수를 파라미터로 사용시 타입이 같아야만 한다 */
func add(_ a: Int, _ b: Int) -> Int {
return a + b
}
func subtract(_ a: Int, _ b: Int) -> Int {
return a - b
}
1. 함수를 변수로 사용
var function = add
function(4, 2) //6
function = subtract
function(4, 2) //2
2. 함수를 파라미터로 사용 : 동일 타입함수끼리 사용 가능
func printResult(_ function: (Int, Int) -> Int, _ a: Int, b: Int) {
let result = function(a, b)
print(result)
}
printResult(add, 10, b: 5)
printResult(subtract, 10, b: 5)
" optional "
" optional "
optional : 변수뒤에 ?를 넣음으로써 nil값도 저장이 가능한 변수타입
/* 옵셔널 optional */
/* 변수 뒤에 ?를 넣음으로서 값이 있을수도 있고 없을수도(nil) 있다는 뜻 */
var carName: String? = "Tesla"
carName = nil
carName = "탱크"
// 과제1. 최애 음식 이름을 담는 변수 (String?)
var favoriteFood: String? = "치킨"
타입을 변환하여 값을 저장하는 경우 nil 값을 포함한 optional 타입으로 저장된다
//string -> Int로 변환이 안되는 경우 nil값으로 저장되는
//optional 변수
// Int?형
let num = Int("10")
" optional - 4가지 기능 "
" optional - forsed unwrapping "
강제로 optional을 없애는 방법 (예 : String? -> String)
-> !를 통해 값을 강제적으로 가져온다
※ 만약 nil값인데 !사용하는 경우 에러가 발생하므로 주의하여 사용해야 한다 ※
var carName: String? = "Tesla"
// forced unwrapping : 강제로 까기
print(carName!) //opstional이 제거되고 값만 출력된다
// 만약 nil 값인경우 error 발생
carName = nil
print(carName!) //error
" optional - binding (if let) "
binding : nil 값 여부에 따라 에러없이 수행하는 방법
var carName: String? = "Tesla"
// optional binding (if let) : 부드럽게 까기 1
if let unwrappedCarName = carName {
print(unwrappedCarName) //nil 값이 아닌경우 수행된다
} else {
print("Car Name 없다") //nil 값인 경우 수행된다
}
// optional binding (if let) : 부드럽게 까지 1
func printParsedInt(from: String) {
if let parsedInt = Int(from) {
print(parsedInt) //nil 값이 아닌 경우 수행된다
} else {
print("Int로 컨버팅 안된다") //nil 값인 경우 수행된다
}
}
printParsedInt(from: "100") //print(parseInt) 수행
printParsedInt(from: "hello") //print("Int로 컨버팅 안된다") 수행
// 과제2. 옵셔널 바인딩을 이용해서 값을 확인해보기
var favoriteFood: String? = "치킨"
if let foodName = favoriteFood {
print(foodName) //nil 값이 아닌경우 수행
} else {
print("좋아하는 음식 없음") //nil 값인 경우 수행
}
" optional - binding (guard) "
binding : nil 값 여부에 따라 에러없이 수행하는 방법
if let 방법 대신에 좀더 깔끔한 코드로 보이게 하는 방법 (효과는 if let과 동일)
// optional binding (guard) : 부드럽게 까기 2
func printParsedInt2(from: String) {
guard let parsedInt = Int(from) else {
print("Int로 컨버팅 안된다.")
return
}
print(parsedInt)
}
printParsedInt2(from: "100") //print(parsedInt) 수행
printParsedInt2(from: "hello") //print("Int로 컨버팅 안된다.") 수행
// 과제3. 닉네임을 받아서 출력하는 함수 만들기, 조건 입력 파라미터는 String?
func printNickname(name: String?) {
guard let NAME = name else {
print("닉네임이 없다!")
return
}
print("닉네임: \(NAME)")
}
printNickname(name: nil) //print("닉네임이 없다!") 수행
printNickname(name: "fdee") //print("닉네임: \(NAME)") 수행
" optional - nil coalescing "
nil coalescing : 변수값 설정시 nil값 대신 초기값을 설정하는 방법
-> ?? 뒤에 초기값을 적는다
// nil coalescing : 값이 없으면 디폴트 값으로 설정
let myCarName: String = carName ?? "model S"
'iOS 개발자 > swift 기초' 카테고리의 다른 글
스위프트 기초6 (structure, method, property, protocol, mutating, extension) (0) | 2021.02.19 |
---|---|
스위프트 기초5 (collection, closure, first class type) (0) | 2021.02.15 |
스위프트 기초4 (collection, array, dictionary, set) (0) | 2021.02.15 |
스위프트 기초2 (while, repeat, for, switch) (0) | 2021.02.09 |
스위프트 기초1 (var, let, if, else, comment, tuple, scope, 3항연산자) (0) | 2021.02.09 |