[ 준비과정 ]
1. java 설치하기 - 구글링
-> terminal 에서 "java -version", "javac -version" 입력시 정상출력 확인
2. eclipse 설치하기 - 구글링
3. postgreSql 설치하기
PostgreSQL: Downloads
Downloads PostgreSQL Downloads PostgreSQL is available for download as ready-to-use packages or installers for various platforms, as well as a source code archive if you want to build it yourself. Packages and Installers Select your operating system family
www.postgresql.org
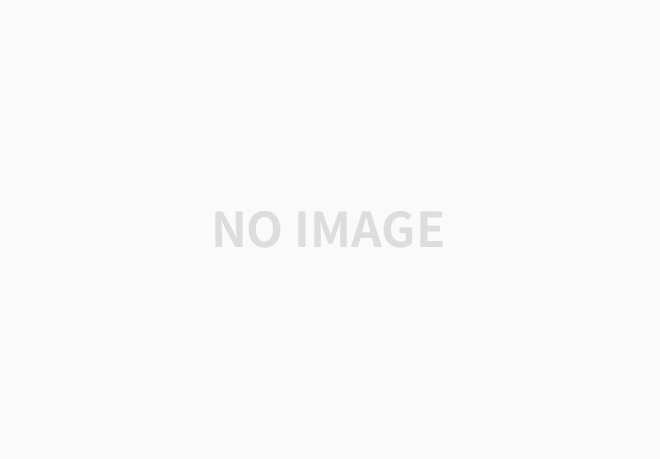
※설치 중간과정 중 비밀번호 꼭 기억하기 ※
설치 완료 후 실행된 모습
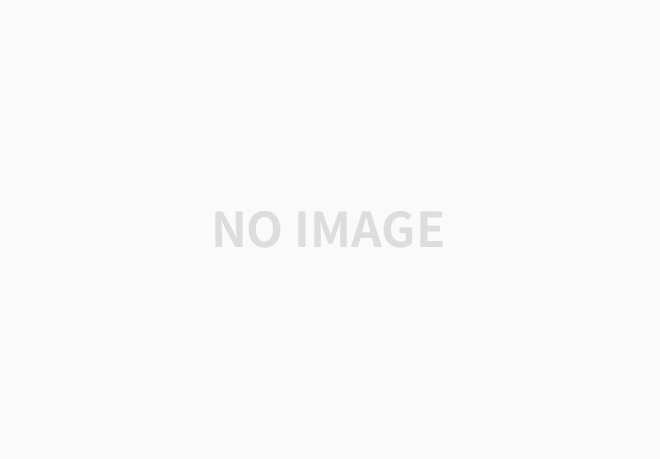
4. postgreSql driver 다운
jdbc.postgresql.org/download.html
PostgreSQL JDBC Download
Download About Binary JAR file downloads of the JDBC driver are available here and the current version with Maven Repository. Because Java is platform neutral, it is a simple process of just downloading the appropriate JAR file and dropping it into your cl
jdbc.postgresql.org
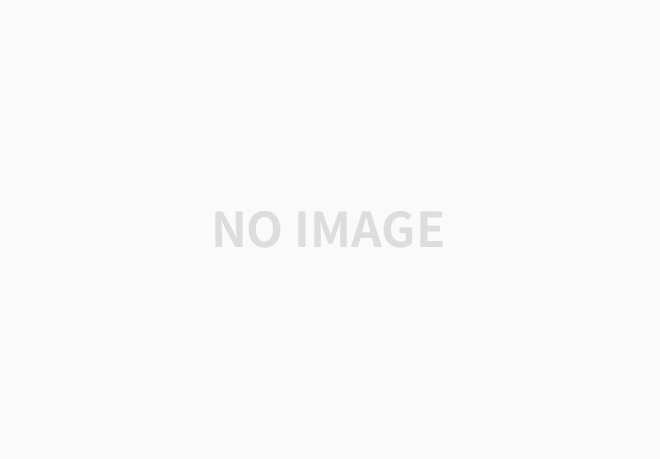
jar 파일이 저장된다
[ eclipse ] - postgreSql의 DB와 연결하는 방법
1. 프로젝트 생성 : New -> Java Project -> Project name 입력
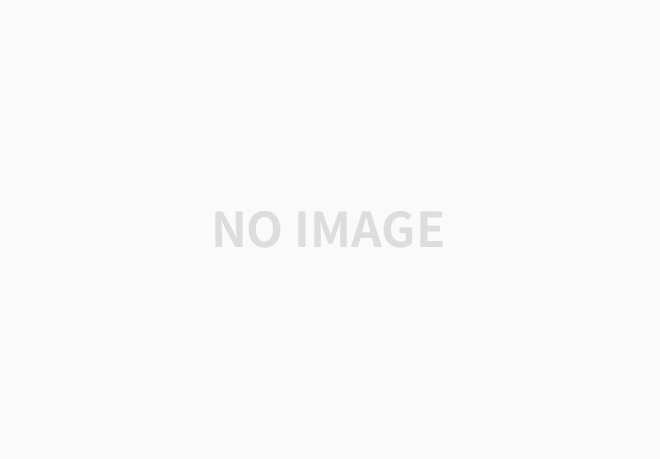
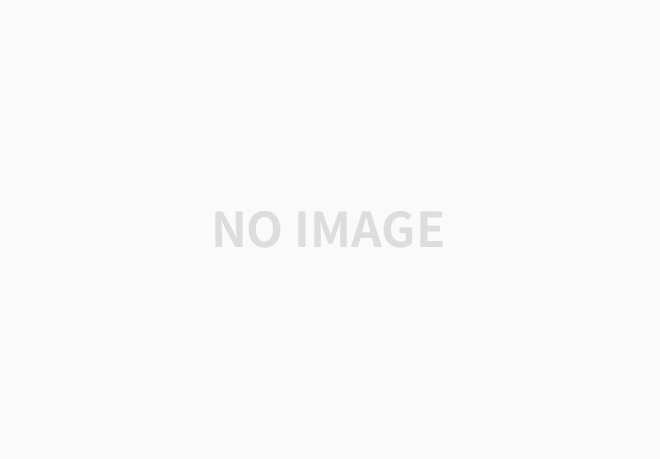
2. Path에 jar 추가 : 프로젝트 우클릭 -> Properties -> Java Build Path -> Libraries -> Modulepath -> Add Library -> User Library -> User Libraries -> New -> 구별할 이름 넣기 (jdbc) -> ok -> 생성된 library 클릭 -> Add External JARs -> jar 파일 클릭 -> Apply and Close -> 새로 만들어진 Library 클릭 -> Finish -> Apply and Close
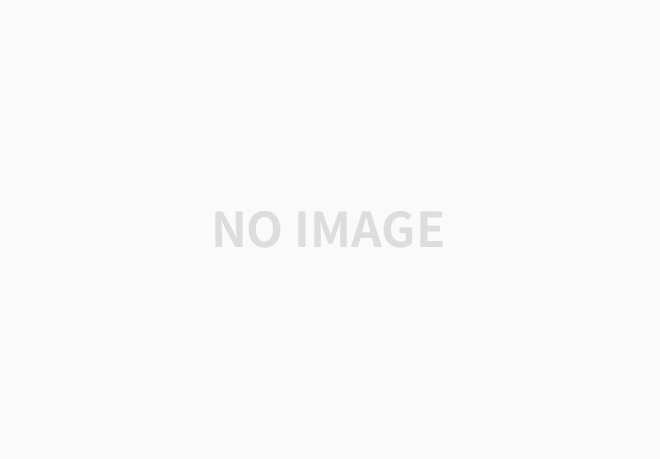
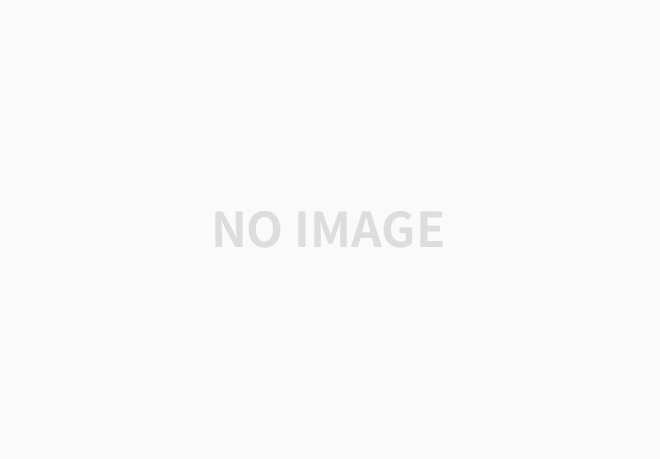
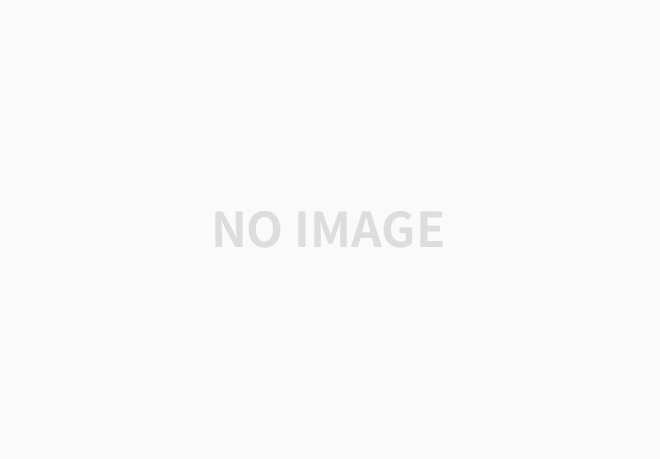
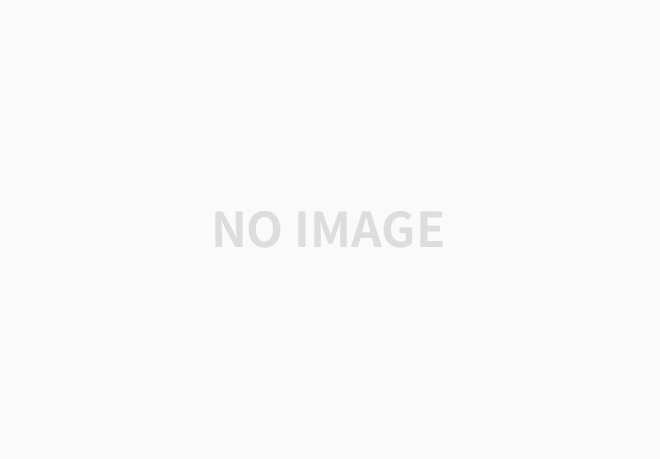
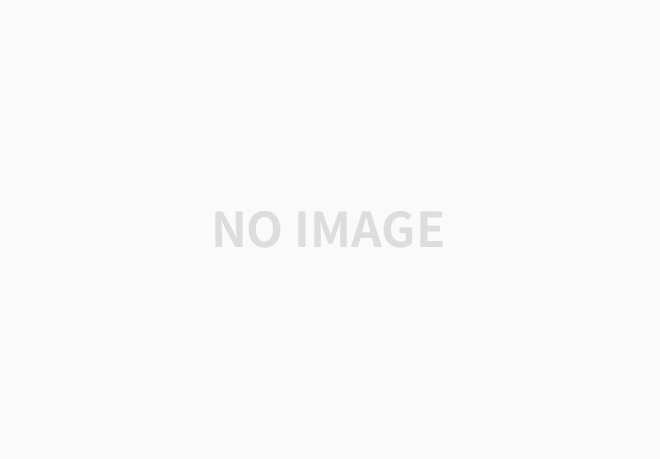
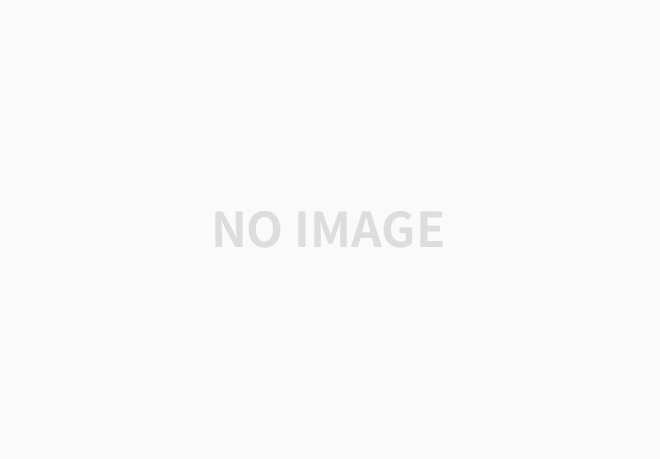
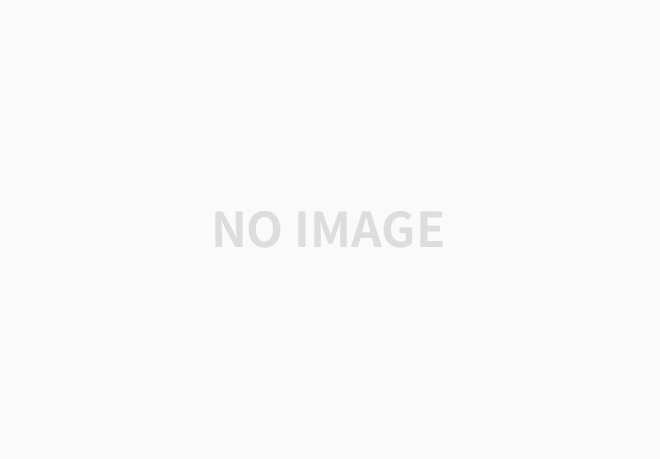
jar 파일 선택
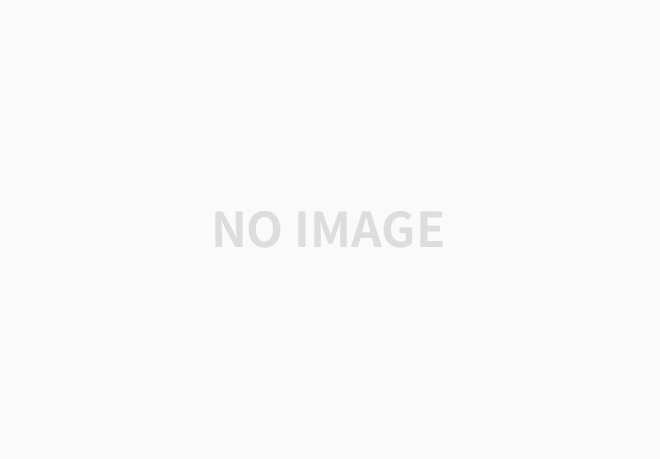
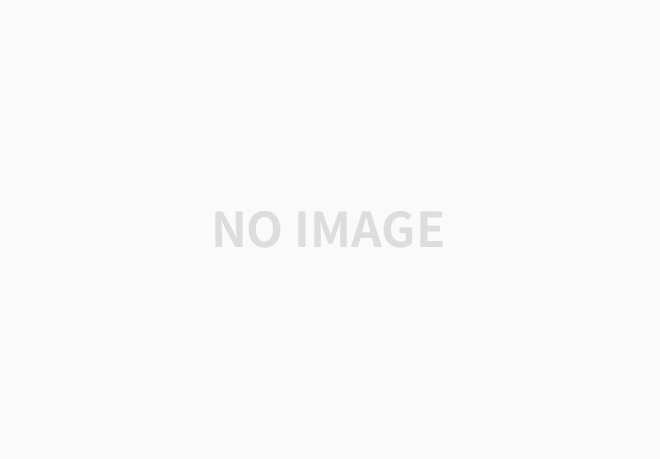
Apply and Close 하여 완료
위 순서에 맞추어 jar 파일이 정상적으로 추가되면 아래와 같이 jar 파일이 추가된다
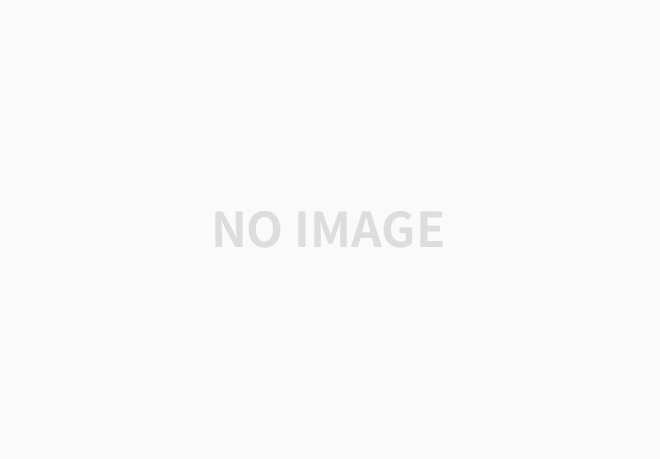
3. Main Class 생성 : 프로젝트 우클릭 -> New -> Class -> 이름 Main 입력
4. JDBC 연결 기본 구조 소스코드
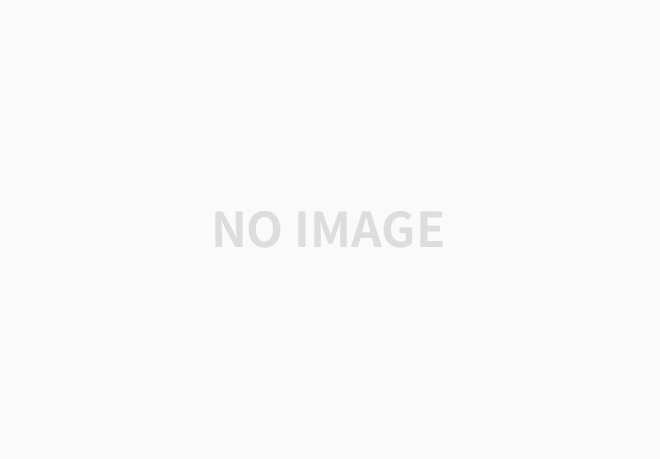
import java.sql.*;
public class Main {
public static void main(String[] args) throws Exception {
try {
Class.forName("org.postgresql.Driver");
} catch (ClassNotFoundException e) {
System.out.println("Where is your PostgreSQL JDBC Driver? Include in your library path!");
e.printStackTrace();
return;
}
System.out.println("PostgreSQL JDBC Driver Registered!");
/// if you have a error in this part, check jdbc driver(.jar file)
Connection connection = null;
try {
connection = DriverManager.getConnection(
"jdbc:postgresql://127.0.0.1:5432/project_movie", "postgres", "cse3207");
} catch (SQLException e) {
System.out.println("Connection Failed! Check output console");
e.printStackTrace();
return;
}
/// if you have a error in this part, check DB information (db_name, user name, password)
if (connection != null) {
System.out.println(connection);
System.out.println("You made it, take control your database now!");
} else {
System.out.println("Failed to make connection!");
}
/////////////////////////////////////////////////////
////////// write your code on this ////////////
/////////////////////////////////////////////////////
connection.close();
}
}
[ eplipse ] - qeury 문 작성방법
CREATE, UPDATE, DELETE 를 위한 변수
Statement st = connection.createStatement();
CREATE TABLE
//1. create table
//st.executeUpdate("퀴리문 작성");
st.executeUpdate("create table department\n"
+ " (dept_name varchar(20), \n"
+ " building varchar(15), \n"
+ " budget numeric(12,2) check (budget > 0),\n"
+ " primary key (dept_name)\n"
+ " )");
INSERT DATA
//2. insert data
//st.executeUpdate("퀴리문 작성");
st.executeUpdate("insert into department values ('Biology', 'Watson', '90000')");
st.executeUpdate("insert into department values ('Comp. Sci.', 'Taylor', '100000')");
DELETE DATA
//3. delete
//st.executeUpdate("퀴리문 작성");
st.executeUpdate("delete from department where dept_name = 'Biology'");
SELECT DATA
DB 내용을 출력하기 위한 변수
//Statement st = connection.createStatement(); 미리 선언
//ResultSet rs = st.executeQuery("쿼리문");
ResultSet rs = st.executeQuery("select * from department");
SELECT DATA 및 PRINT DATA
//4. selection
ResultSet rs = st.executeQuery("select * from department");
//DB의 각 attribute들
String dept_name;
String building;
Float budget;
while(rs.next()) {
dept_name = rs.getString(1);
building = rs.getString(2);
budget = rs.getFloat(3);
System.out.println(dept_name+", "+building+", "+budget);
}
NULL 값인지 확인
int a = rs.getInt("a");
if(rs.wasNull()) Systems.out.println("Got null value");
query문 형식을 미리 설정
PreparedStatement pStmt = conn.prepareStatement("insert into instructor values(?,?,?,?)");
pStmt.setString(1, "88877");
pStmt.setString(2, "Perry");
pStmt.setString(3, "Finance");
pStmt.setString(4, "125000");
pStmt.executeUpdate();
pStmt.setString(1, "88878")
pStmt.executeUpdate();
'DB (DataBase)' 카테고리의 다른 글
쿼리문 임시저장소 (0) | 2021.04.12 |
---|---|
SQL 쿼리문 정리 - Subquery (0) | 2021.04.07 |
SQL 쿼리문 정리 (0) | 2021.04.06 |
SQL 쿼리문 정리 - BASIC (0) | 2021.04.05 |